Automation of testing using multiple devices with shell scripts
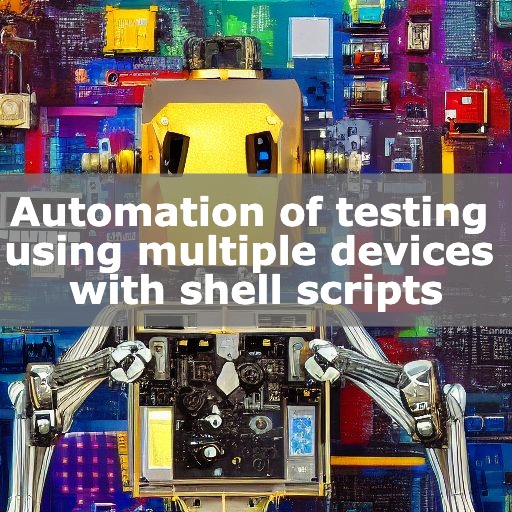
We believe that everyone is implementing test automation.
If you want to automate a combined or comprehensive test that straddles multiple devices rather than a single device, you can do so flexibly with shell scripts, which we will introduce here.
Login with Script
Create a shell script to login to the terminal to be connected via ssh.
For the public cloud, the key is specified with '-i’ to log in using a key.
Also, it is common to leave fingerprints when logging in, but an error occurs when logging in with VIP, so the 'StrictHostKeyChecking no’ option is specified to ignore fingerprints.
In addition, the '-t’ option allows the command to be executed as a pseudo-terminal.
◆login.sh
#!/bin/bash
sudo ssh -i ~/.ssh/id_rsa -o 'StrictHostKeyChecking no' -t user@x.x.x.x
You can log in by actually running the script you created to see if it is usable.
% sh login.sh
Create the script you want to run
Create a script that you want to run.
Here we simply obtain the date and time.
◆work.sh
#!/bin/bash
date >> test_work.txt
date
exit
Execute shell script
Prepare a work.sh script that describes what you want to execute. Pass this script file at login.
After multiple shell executions, download the file that outputs the execution results and receive the results.
◆auto.sh
sh login.sh 'sh' < work.sh
sudo scp -P 22 -i ~/.ssh/id_rsa user@x.x.x.x:/home/user/test_work.txt ./
When executed, the file can be retrieved automatically.
sh auto.sh
You can also run multiple shells simultaneously.
By running them in the background (&) and waiting for the process to finish, you can make simultaneous connections via ssh.
◆auto2.sh
sh login.sh 'sh' < work.sh &
sh login2.sh 'sh' < work.sh &
wait
sudo scp -P 22 -i ~/.ssh/id_rsa user@x.x.x.x:/home/user/test_work.txt ./
sudo scp -P 22 -i ~/.ssh/id_rsa user@y.y.y.y:/home/user/test_work.txt ./
Execute shell scripts.
sh auto2.sh
Logging by Script
Here is an example of how to apply this to access and log the web while retrieving log files.
The following script will retrieve a new Nginx access log for 10 seconds.
◆work2.sh
(sleep 10 ;kill $$) &
exec sudo tail -n 0 /var/log/nginx/access.log > ~/access.log
◆auto3.sh
While accessing with work2.sh, you can automate the sequence of accessing from your own terminal with curl and acquiring logs, so that you can perform the test.
sh login.sh 'sh' < work2.sh &
curl http://x.x.x.x
wait
sudo scp -P 22 -i ~/.ssh/id_rsa user@x.x.x.x:/home/user/access.log ./
This can be done as follows.
sh auto3.sh
Examination of results
If the end result is to compare the results with the expected values, I believe the test can be automated by compiling the downloaded log files into Excel and comparing them in Excel.